JavaSE-5进阶线程单元测试反射注解XML
1. 单元测试
1.1 单元测试
1.1.1 单元测试概述
1.1.2 Junit单元测试框架
1.1.3 单元测试快速入门——步骤
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package com.curious.javasepro.d21_junit;
public class UserService { public String loginName(String loginName, String passWord) { if ("admin".equals(loginName) && "123456".equals(passWord)) { return "登陆成功"; } else { return "用户名或密码有问题"; } }
public void selectName() { System.out.println(10/0); System.out.println("查询全部用户名称成功~~"); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.curious.javasepro.d21_junit;
import org.junit.Assert; import org.junit.Test;
public class TestUserService {
@Test public void testLoginName() { UserService userService = new UserService(); String res = userService.loginName("admin", "123456");
Assert.assertEquals("您的功能业务数据可能出现bug", "登陆成功", res); } }
|

1 2 3 4 5
| @Test public void testSelectNames() { UserService userService = new UserService(); userService.selectName(); }
|

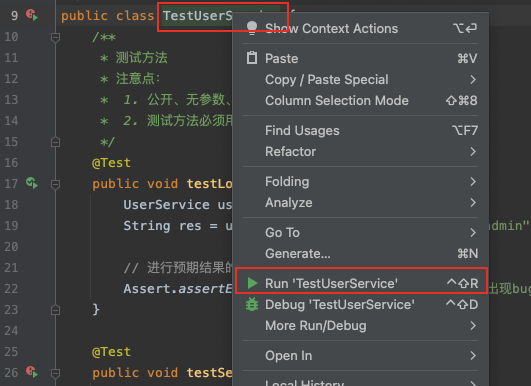

1.1.4 单元测试常用注解
- 开始执行的方法:初始化资源
- 执行完之后的方法:释放资源
2. 反射(重点)
2.1 反射概述
2.2 反射的第一步:获取Class类的对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| package com.curious.javasepro.d22_reflect;
public class Test { public static void main(String[] args) throws Exception { Class c = Class.forName("com.curious.javasepro.d22_reflect.Student"); System.out.println(c);
Class c1 = Student.class; System.out.println(c1);
Student s = new Student(); Class c2 = s.getClass(); System.out.println(c2); } }
|
2.3 反射获取构造器对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| package com.curious.javasepro.d22_reflect_constructor;
public class Student { private String name; private int age;
private Student() {
}
public Student(String name, int age) { this.name = name; this.age = age; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
@Override public String toString() { return "Student{" + "name='" + name + '\'' + ", age=" + age + '}'; }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| package com.curious.javasepro.d22_reflect_constructor;
import org.junit.Test;
import java.lang.reflect.Constructor;
public class TestStudent01 { @Test public void getConstructors() { Class c = Student.class; Constructor[] constructors = c.getConstructors(); for (Constructor constructor: constructors) { System.out.println(constructor.getName() + " ====> " + constructor.getParameterCount()); } }
@Test public void getDeclaredConstructors() { Class c = Student.class; Constructor[] constructors = c.getDeclaredConstructors(); for (Constructor constructor: constructors) { System.out.println(constructor.getName() + " ====> " + constructor.getParameterCount()); } }
@Test public void getConstructor() throws Exception { Class c = Student.class; Constructor cons = c.getConstructor(String.class, int.class); System.out.println(cons.getName() + " ====> " + cons.getParameterCount());
}
@Test public void getDeclaredConstructor() throws Exception { Class c = Student.class; Constructor cons = c.getDeclaredConstructor(); System.out.println(cons.getName() + " ====> " + cons.getParameterCount());
} }
|
2.4 使用反射获取的构造器对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| package com.curious.javasepro.d22_reflect_constructor;
import org.junit.Test;
import java.lang.reflect.Constructor;
public class TestStudent02 { @Test public void getDeclaredConstructor() throws Exception { Class c = Student.class;
Constructor cons = c.getDeclaredConstructor();
System.out.println(cons.getName() + " ====> " + cons.getParameterCount());
cons.setAccessible(true); Student s = (Student) cons.newInstance(); System.out.println(s);
Constructor cons1 = c.getDeclaredConstructor(String.class, int.class); Student s1 = (Student) cons1.newInstance("ABC", 12); System.out.println(s1);
} }
|
Student{name=’null’, age=0}
Student{name=’ABC’, age=12}
2.5 反射获取成员变量对象
第一步拿到类对象xxx.class,例如Student.class
相当于拿到所有的代码
2.6 使用反射获取的成员变量对象
2.7 使用反射获取方法对象
- 获取+使用(DeclaredMethods,把私有的也暴力搞出来)
2.8 使用反射获取的方法对象
2.9 反射的作用——绕过编译阶段为集合添加数据、做企业级通用框架
2.9.1 绕过编译阶段为集合添加数据
2.9.2 通用框架的底层原理
只有反射才能解决这个场景的问题↑
3. 注解
3.1 注解概述、作用
3.2 自定义注解
3.3 元注解
3.4 注解解析
3.4.1 注解的解析与技巧
3.4.2 注解解析的案例
3.5 注解的应用:模拟junit框架
有注解,使用反射机制触发执行
测试必须无参数无返回值
4. 动态代理(重难点)
4.1 代理概述,无代理的场景
这里还有一种解释是nginx的反向代理,还有VPN的正向代理
对对象的行为做一些额外的操作
4.2 如何创建代理对象
调用s2.jump()
,走的流程
未来:对象给Spring,然后返回一个更强大的对象
4.3 动态代理的应用案例(理解使用动态代理的优势)
动态代理必须基于接口进行设计
public class UserServiceImpl implements UserService
上面重复代码太多了,前面要干一件事,后面要干一件事,这样用代理提高代码复用性,就不用每次都写一下这个时间统计了
4.4 动态代理的优点
泛型的进一步优化
5. XML文件(简单了解,未来有接触到再进一步学习)
5.1 XML概述
5.2 语法规则
5.3 文档约束(文档需要定统一的规则)
5.4 XML解析
5.5 其他规则,未来使用时候进一步学习了
6. 设计模式补充
6.1 工厂模式
只从工厂这一个地方来改就行了,类似个配置文件?
6.2 装饰模式
一个方法的功能,知识为了增强另一个类的功能